2021-09-24 14:19:51 +02:00
|
|
|
# workgroups
|
|
|
|
|
2021-09-27 10:46:34 +02:00
|
|
|
Package workgroups is a little helper for creating workers
|
2021-09-27 11:06:57 +02:00
|
|
|
with the help of [sync.Errgroup](https://pkg.go.dev/golang.org/x/sync/errgroup).
|
2021-09-27 10:46:34 +02:00
|
|
|
|
|
|
|

|
|
|
|

|
|
|
|

|
|
|
|
|
2021-09-27 11:03:38 +02:00
|
|
|
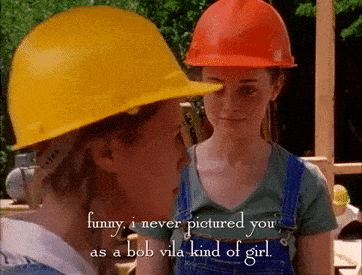
|
|
|
|
|
|
|
|
## Links
|
|
|
|
|
|
|
|
* [build](https://ci.xsfx.dev/xsteadfastx/workgroups)
|
|
|
|
* [coverage](https://codecov.io/github/xsteadfastx/workgroups/)
|
|
|
|
* [report](https://goreportcard.com/report/go.xsfx.dev/workgroups)
|
|
|
|
* [reference](https://pkg.go.dev/go.xsfx.dev/workgroups)
|
2021-09-27 10:46:34 +02:00
|
|
|
|
|
|
|
## Variables
|
|
|
|
|
|
|
|
ErrInWorker is an error that gets returned if there is a error
|
|
|
|
in the work function.
|
|
|
|
|
|
|
|
```golang
|
|
|
|
var ErrInWorker = errors.New("received error in worker")
|
|
|
|
```
|
|
|
|
|
|
|
|
## Types
|
|
|
|
|
2021-09-27 11:03:38 +02:00
|
|
|
### type [Dispatcher](/workgroups.go#L52)
|
2021-09-27 10:46:34 +02:00
|
|
|
|
|
|
|
`type Dispatcher struct { ... }`
|
|
|
|
|
|
|
|
Dispatcher carries the job queue, the errgroup and the number of workers
|
|
|
|
to start.
|
|
|
|
|
2021-09-27 11:03:38 +02:00
|
|
|
#### func (*Dispatcher) [Append](/workgroups.go#L102)
|
2021-09-27 10:46:34 +02:00
|
|
|
|
|
|
|
`func (d *Dispatcher) Append(job Job)`
|
|
|
|
|
|
|
|
Append adds a job to the work queue.
|
|
|
|
|
2021-09-27 11:03:38 +02:00
|
|
|
#### func (*Dispatcher) [Close](/workgroups.go#L108)
|
2021-09-27 10:46:34 +02:00
|
|
|
|
|
|
|
`func (d *Dispatcher) Close()`
|
|
|
|
|
|
|
|
Close closes the queue channel.
|
|
|
|
|
2021-09-27 11:03:38 +02:00
|
|
|
#### func (*Dispatcher) [Start](/workgroups.go#L71)
|
2021-09-27 10:46:34 +02:00
|
|
|
|
|
|
|
`func (d *Dispatcher) Start()`
|
|
|
|
|
|
|
|
Start starts the configured number of workers and waits for jobs.
|
|
|
|
|
2021-09-27 11:06:57 +02:00
|
|
|
#### func (*Dispatcher) [Wait](/workgroups.go#L114)
|
2021-09-27 10:46:34 +02:00
|
|
|
|
|
|
|
`func (d *Dispatcher) Wait() error`
|
|
|
|
|
2021-09-27 11:06:57 +02:00
|
|
|
Wait for all jobs to finnish.
|
|
|
|
|
2021-09-27 11:03:38 +02:00
|
|
|
### type [Job](/workgroups.go#L40)
|
2021-09-27 10:46:34 +02:00
|
|
|
|
|
|
|
`type Job struct { ... }`
|
|
|
|
|
|
|
|
Job carries a job with everything it needs.
|
|
|
|
I know know that contexts shouldnt be stored in a struct.
|
|
|
|
Here is an exception, because its a short living object.
|
|
|
|
The context is only used as argument for the Work function.
|
|
|
|
Please use the NewJob function to get around this context in struct shenanigans.
|
|
|
|
|
2021-09-27 11:03:38 +02:00
|
|
|
### type [Work](/workgroups.go#L33)
|
2021-09-27 10:46:34 +02:00
|
|
|
|
|
|
|
`type Work func(ctx context.Context) error`
|
|
|
|
|
|
|
|
Work is a type that defines worker work.
|
|
|
|
|
|
|
|
## Examples
|
|
|
|
|
|
|
|
```golang
|
|
|
|
package main
|
|
|
|
|
|
|
|
import (
|
|
|
|
"context"
|
|
|
|
"fmt"
|
|
|
|
"go.xsfx.dev/workgroups"
|
|
|
|
"log"
|
|
|
|
"runtime"
|
|
|
|
)
|
|
|
|
|
|
|
|
func main() {
|
|
|
|
d, ctx := workgroups.NewDispatcher(
|
|
|
|
context.Background(),
|
|
|
|
runtime.GOMAXPROCS(0), // This starts as much worker as maximal processes are allowed for go.
|
2021-10-07 13:55:22 +02:00
|
|
|
10, // Capacity of the queue.
|
2021-09-27 10:46:34 +02:00
|
|
|
)
|
|
|
|
|
|
|
|
work := func(ctx context.Context) error {
|
|
|
|
// Check if context already expired.
|
|
|
|
// Return if its the case, else just go forward.
|
|
|
|
select {
|
|
|
|
case <-ctx.Done():
|
|
|
|
return fmt.Errorf("got error from context: %w", ctx.Err())
|
|
|
|
default:
|
|
|
|
}
|
|
|
|
|
|
|
|
// Some wannebe work... printing something.
|
|
|
|
fmt.Print("hello world from work")
|
|
|
|
|
|
|
|
return nil
|
|
|
|
}
|
|
|
|
|
|
|
|
// Starting up the workers.
|
|
|
|
d.Start()
|
|
|
|
|
|
|
|
// Feeding the workers some work.
|
|
|
|
d.Append(workgroups.NewJob(ctx, work))
|
|
|
|
|
|
|
|
// Closing the channel for work.
|
|
|
|
d.Close()
|
|
|
|
|
|
|
|
// Waiting to finnish everything.
|
|
|
|
if err := d.Wait(); err != nil {
|
|
|
|
log.Fatal(err)
|
|
|
|
}
|
|
|
|
|
|
|
|
}
|
|
|
|
|
|
|
|
```
|
|
|
|
|
|
|
|
Output:
|
|
|
|
|
|
|
|
```
|
|
|
|
hello world from work
|
|
|
|
```
|